コンソールアプリで実行ファイルのディレクトリのパス、ディレクトリ名を取得する - C#
コンソールアプリで実行ファイルのディレクトリのパス、ディレクトリ名を取得するコードを紹介します。
概要
こちらの記事では、Windows Formアプリケーションで、アプリケーションの実行ディレクトリを取得するコードを紹介しました。
紹介した方法では、
System.Windows.Forms.Application
オブジェクトを利用するため、コンソールアプリケーションで同様の処理を実行する場合は、
System.Windows.Forms をアプリケーションの参照に追加する必要があり、冗長になってしまいます。
この記事では、Applicationoオブジェクトを利用しない方法で実行ファイルのディレクトリのパスを取得するコードを紹介します。
方法
Applicationオブジェクトを利用せずに、実行時のパスを取得する方法には以下があります。
- Assembly.GetEntryAssembly() メソッドを利用する
- Assembly.GetExecutingAssembly() メソッドを利用する
- AppDomain.CurrentDomain.SetupInformation.ApplicationBase プロパティを利用する
- Environment.CommandLine プロパティを利用する
- Environment.GetCommandLineArgs() を利用し最初の引数を取得する
プログラム
コンソールアプリケーションを作成し、以下のコードを作成します。
コード
// See https://aka.ms/new-console-template for more information
using System.Reflection;
string entry_assembly_path = Assembly.GetEntryAssembly().Location;
string executing_assembly_path = Assembly.GetExecutingAssembly().Location;
string app_base_path = AppDomain.CurrentDomain.SetupInformation.ApplicationBase;
string comm_path = Environment.CommandLine;
string args_path = Environment.GetCommandLineArgs()[0];
Console.WriteLine("GetEntryAssembly: "+entry_assembly_path);
Console.WriteLine("GetExecutingAssembly: " + executing_assembly_path);
Console.WriteLine("ApplicationBase: " + app_base_path);
Console.WriteLine("CommandLine: " + comm_path);
Console.WriteLine("GetCommandLineArgs: " + args_path);
Console.ReadLine();
using System;
using System.Reflection;
namespace GetPathConsoleClassic
{
internal class Program
{
static void Main(string[] args)
{
string entry_assembly_path = Assembly.GetEntryAssembly().Location;
string executing_assembly_path = Assembly.GetExecutingAssembly().Location;
string app_base_path = AppDomain.CurrentDomain.SetupInformation.ApplicationBase;
string comm_path = Environment.CommandLine;
string args_path = Environment.GetCommandLineArgs()[0];
Console.WriteLine("GetEntryAssembly: "+entry_assembly_path);
Console.WriteLine("GetExecutingAssembly: " + executing_assembly_path);
Console.WriteLine("ApplicationBase: " + app_base_path);
Console.WriteLine("CommandLine: " + comm_path);
Console.WriteLine("GetCommandLineArgs: " + args_path);
Console.ReadLine();
}
}
}
解説
Assembly.GetEntryAssembly() によりプロセスのアセンブリ情報を取得できます。
Location
プロパティに実行ファイルのパスが設定されています。
Assembly.GetExecutingAssembly()により、現在実行中のアセンブリ情報を取得できます。
Location
プロパティに実行ファイルのパスが設定されています。
AppDomain.CurrentDomain.SetupInformation.ApplicationBase プロパティにアプリケーションを含むディレクトリの名前が設定されています。
Environment.CommandLine にプログラム名とコマンドラインに指定されたすべてのパラメーターを取得できます。
Environment.GetCommandLineArgs()[0] には、コマンドラインの最初のパラメーターである実行ファイルのパスが格納されています。
実行結果
.NET コンソールアプリケーション
.NET 6.0のコンソールアプリケーションでの実行結果です。
.NET の場合は、コンソールアプリケーションのexeファイルではなく、dllファイルが実行ファイルのパスとして表示されます。
実行時にパラメーターが設定されている場合の結果です。CommandLine プロパティにはパラメーターの文字列も含まれていることが確認できます。
.NET Framework コンソールアプリケーション
.NET Framework 4.8のコンソールアプリケーションでの実行結果です。
.NET Frameworkの場合は、コンソールアプリケーションのexeファイルのパスが実行プログラムのパスとして取得できます。
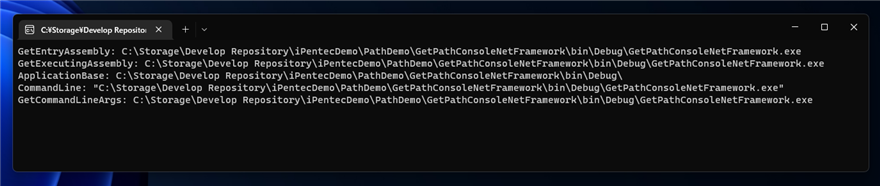
実行時にパラメーターが設定されている場合の結果です。CommandLine プロパティにはパラメーターの文字列も含まれていることが確認できます。
著者
iPentecのメインプログラマー
C#, ASP.NET の開発がメイン、少し前まではDelphiを愛用