オブジェクトの配列形式のJSONを書き出す - C#
配列形式のJSONファイルを書き出すコードを紹介します。
プログラム例
UI
下図のフォームをデザインします。フォームにボタンを1つ配置します。
コード
下記のコードを記述します。
#
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
using System.Runtime.Serialization.Json;
namespace JsonArraySerializeDemo
{
public partial class FormMain : Form
{
public FormMain()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
MyItem[] items = new MyItem[5];
items[0] = new MyItem();
items[0].Id = 0;
items[0].Name = "Penguin";
items[0].Color = "navy";
items[1] = new MyItem();
items[1].Id = 1;
items[1].Name = "Owl";
items[1].Color = "gray";
items[2] = new MyItem();
items[2].Id = 0;
items[2].Name = "Giraffe";
items[2].Color = "yellow";
items[3] = new MyItem();
items[3].Id = 0;
items[3].Name = "White Bear";
items[3].Color = "white";
items[4] = new MyItem();
items[4].Id = 0;
items[4].Name = "Flamingo";
items[4].Color = "pink";
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(MyItem[]));
FileStream fs = new FileStream("output.json", FileMode.Create);
try {
serializer.WriteObject(fs, items);
}
finally {
fs.Close();
}
}
}
}
#
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Runtime.Serialization;
namespace JsonArraySerializeDemo
{
[System.Runtime.Serialization.DataContract]
public class MyItem
{
[System.Runtime.Serialization.DataMember()]
public int Id;
[System.Runtime.Serialization.DataMember()]
public string Name;
[System.Runtime.Serialization.DataMember()]
public string Color;
}
}
解説
JSONの書き出しの基本については
こちらの記事を参照してください。
FormMain.cs
下記のコードでクラスのインスタンスを作成し、書き出す情報をクラスオブジェクトに設定します。
MyItem[] items = new MyItem[5];
items[0] = new MyItem();
items[0].Id = 0;
items[0].Name = "Penguin";
items[0].Color = "navy";
items[1] = new MyItem();
items[1].Id = 1;
items[1].Name = "Owl";
items[1].Color = "gray";
items[2] = new MyItem();
items[2].Id = 0;
items[2].Name = "Giraffe";
items[2].Color = "yellow";
items[3] = new MyItem();
items[3].Id = 0;
items[3].Name = "White Bear";
items[3].Color = "white";
items[4] = new MyItem();
items[4].Id = 0;
items[4].Name = "Flamingo";
items[4].Color = "pink";
下記のコードにてクラスをJSON形式でシリアライズしてファイルに書き出しています。
オブジェクトの配列を書き出す場合は、DataContractJsonSerializerの引数ににクラスの配列型を与えます。
またシリアライザーのWriteObjectメソッドにも書き出したいオブジェクトの配列を与えます。
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(MyItem[]));
FileStream fs = new FileStream("output.json", FileMode.Create);
try {
serializer.WriteObject(fs, items);
}
finally {
fs.Close();
}
MyItem.cs
MyItem.csはJSONのオブジェクトのクラスです。"Id","Name","Color"をJSONで書き出す項目として設定しています。
実行結果
プロジェクトを実行します。下図のウィンドウが表示されますので、button1を押します。
ウィンドウを閉じ、実行ファイルのあるディレクトリを開きます。"output.json"ファイルが作成されています。
"output.json"ファイルをメモ帳で開きます。
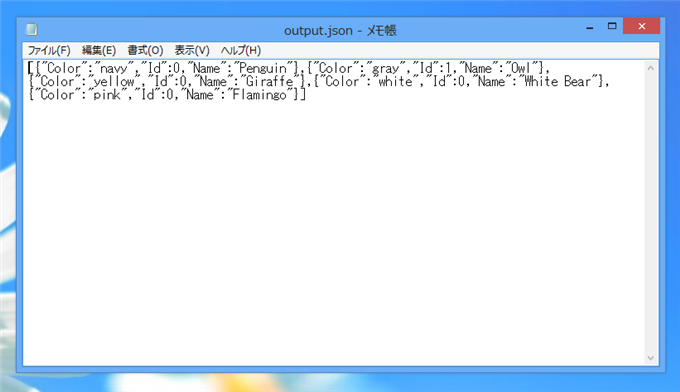
#
[
{"Color":"navy","Id":0,"Name":"Penguin"},
{"Color":"gray","Id":1,"Name":"Owl"},
{"Color":"yellow","Id":0,"Name":"Giraffe"},
{"Color":"white","Id":0,"Name":"White Bear"},
{"Color":"pink","Id":0,"Name":"Flamingo"}
]
著者
iPentecのメインプログラマー
C#, ASP.NET の開発がメイン、少し前まではDelphiを愛用